Editor Series - Configuring Emacs To Fit The Needs Part 2
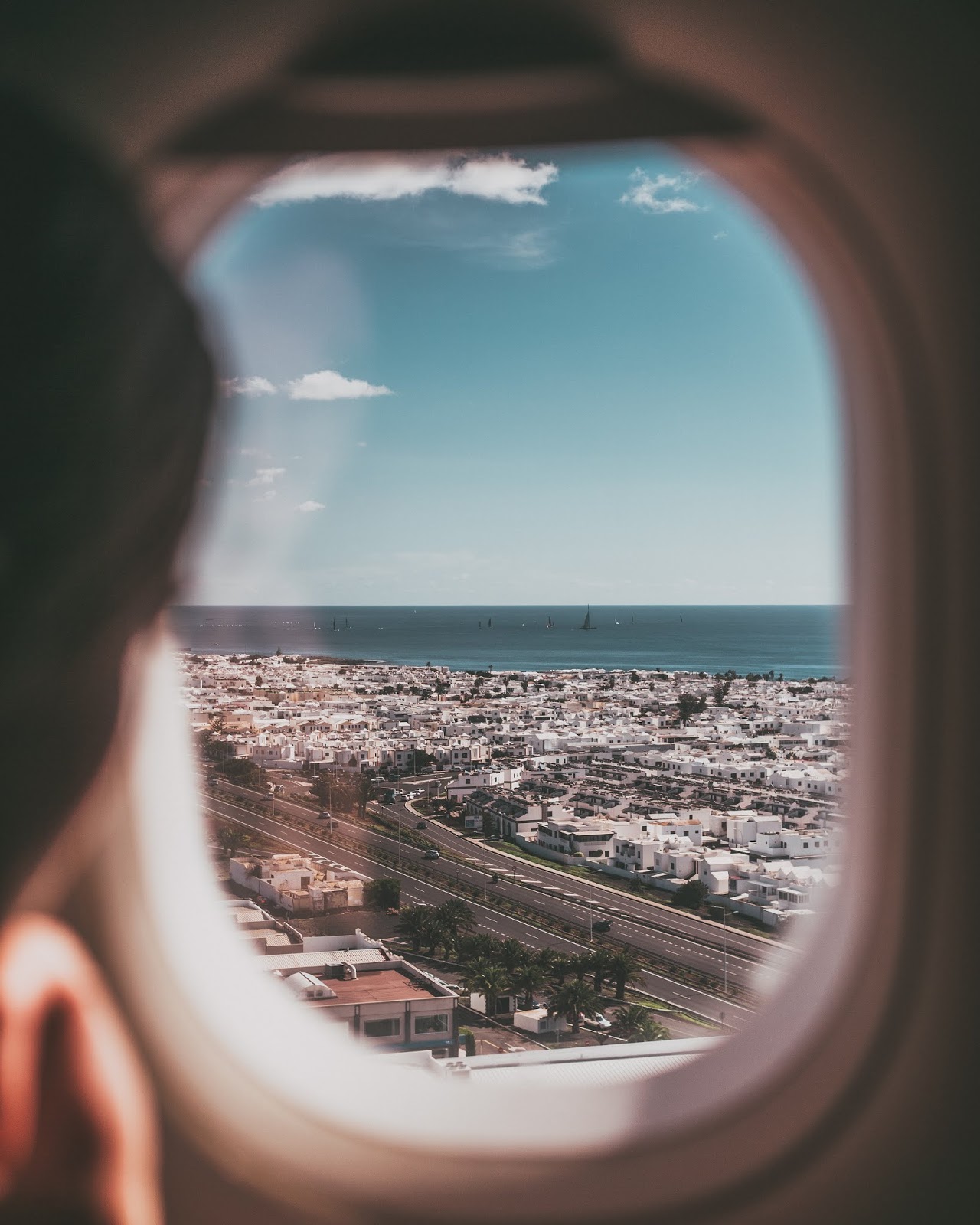
The world as we have created it is a process of our thinking. It cannot be changed without changing our thinking.
— Albert Einstein.
As the title says, to fit the needs. Currently, I’m looking into handling multiple projects but vast differ in programming paradigm and language. Some of the language that I’ll probably tackling are Go, Rust, Ruby, Perl, C++, JavaScript, Typescript, and etc.
Prerequisites
First of all, you need Emacs 26 and up as this is the main topic of this article that we need to configure. There are many ways to install it on your system, kindly check your GNU/Linux distribution for specific ways to install it.
Packages, Oh For The Brave
If its your first time configuring Emacs, then head out first to my first article here. After that, we will establish all the needed packages.
Diminish, Bind and Org
The package diminish
will remove any mode in status bar, while the bind
will map the keys to a specific lisp function. And not to forgot org-mode
, it can be your to-do / agenda list. Actually there are many things you could do with org
, you could even write a book with it.
(use-package diminish)
(use-package bind-key)
(use-package org
:ensure org-plus-contrib
:defer 7)
Uniquify
The uniquify
package will change buffer name instead of file.txt|<1> to something like file.txt|project. A good change specially if you’re constantly using buffers.
(require 'uniquify)
(setq uniquify-buffer-name-style 'forward)
Flycheck
The de facto package for linting, validating, and checking is flycheck
. Almost any Emacs config you can find on the internet contains flycheck
to handle checking.
(use-package flycheck
:defer 5
:diminish flycheck-mode
:hook (after-init . global-flycheck-mode)
:config
(flycheck-define-checker proselint
"A linter for prose."
:command ("proselint" source-inplace)
:error-patterns
((warning line-start (file-name) ":" line ":" column ": "
(id (one-or-more (not (any " "))))
(message) line-end))
:modes (text-mode markdown-mode adoc-mode gfm-mode org-mode))
(add-to-list 'flycheck-checkers 'proselint))
Magit
The magit
package handles diff from head state and it supports many git vcs command. It can query the status of the project directory and see file changes.
(use-package magit
:pin melpa-stable
:defer 1
:init (diminish 'magit-auto-revert-mode)
:hook (magit-status-sections . magit-insert-worktrees)
:config (progn
(setq magit-commit-show-diff nil
magit-last-seen-setup-instructions "1.4.0")))
Multiple Cursors
Multiple cursor mode is to handle selection of all similar words and its also capable of setting multiple cursor at point. This can be very useful when you want to modify a set of words, and in different line / column concurrently.
(use-package multiple-cursors
:bind ("C-S-c C-S-c" . mc/edit-lines))
Helm
The helm
package, super extendable and has many use case. But in short, its more of selection narrowing package. The package can configured to handle several task, using additional extension packages.
(use-package helm
:bind (("M-x" . helm-M-x)
("C-x C-f" . helm-find-files))
:diminish helm-mode
:config (progn
(setq helm-buffers-fuzzy-matching t)
(setq helm-grep-ag-command "rg --color=always --colors 'match:fg:black' --colors 'match:bg:yellow' --smart-case --no-heading --line-number %s %s %s")
(setq helm-grep-ag-pipe-cmd-switches '("--colors 'match:fg:black'" "--colors 'matcnh:bg:yellow'"))
(helm-mode 1)))
Expand Region
The expand-region
package is useful if you want to expand into similar brackets or tags.
Checkout its GitHub repository to know more.
(use-package expand-region
:bind ("C-=" . er/expand-region))
Projectile
If you have many projects then projectile
is for you. It can fuzzy find project and switch to another project easily. I always install this package, as for me its so necessary for project management.
(use-package projectile
:diminish projectile-mode
:config (progn
(projectile-mode +1)
(setq projectile-enable-caching nil)
(setq projectile-completion-system 'helm)))
(use-package helm-projectile
:bind ("M-t" . helm-projectile-find-file)
:config
(helm-projectile-on))
Yasnippet
The yasnippet
is a great tool to make an abbreviated shortcut to insert a specific snippet. Its similar to snippetmate
in vim.
(use-package yasnippet
:ensure t
:diminish yas-minor-mode
:init (yas-global-mode 1))
(use-package yasnippet-snippets
:defer 1
:after (yasnippet))
Which Key
Forgot the keys you want to press? which-key
provides a list of key binds you can press after a specific key map. This makes learning Emacs easy for novice.
(use-package which-key
:defer 1
:diminish which-key-mode
:config
(which-key-mode))
Editor Helpers
These are package that can make editor happy. The yaml-mode
handles yaml type files, while markdown-mode
for markdown files. The editorconfig
respects the bundled editor config in project root.
(use-package yaml-mode)
(use-package markdown-mode
:hook (markdown-mode . visual-line-mode))
(use-package editorconfig
:config
(editorconfig-mode 1))
Undo Tree
Want to undo into a specific state? undo-tree
can easily backtrack changes and jump into a specific state. Its more powerful when combined with Emacs backup.
(use-package undo-tree
:ensure t
:init
(global-undo-tree-mode))
Rainbow Delimiters
Want to have rainbow delimiters, then install this package. It will adjust pair bracket color base on its hierarchical level. Very handy if your programming lisp and Clojure.
(use-package rainbow-delimiters
:hook (prog-mode . rainbow-delimiters-mode))
Avy
The avy
package can be use to jump to a specific character inside the buffer and easily move line to line. Its set to M-g
then c
to jump to a character.
(use-package avy
:ensure t
:bind
("H-SPC" . avy-goto-char-timer)
("H-w" . avy-goto-word-1)
("H-c" . avy-goto-char-2)
("H-l" . avy-goto-line)
("H-d" . avy-goto-word-0)
("<f9> SPC" . avy-goto-char-timer)
("C-c g" . avy-goto-word-1)
("M-g l" . avy-goto-line)
("M-g c" . avy-goto-char-2)
("M-g w" . avy-goto-word-0))
Smartparens
The smartparens
automatically inserts pair bracket or quote. Also can traverse between beginning and end of pair symbol. Works out of the box with html-mode
traversing tags.
(use-package smartparens
:ensure t
:diminish smartparens-mode
:config
(progn
(use-package smartparens-config)
(smartparens-global-mode 1)))
Miscellaneous
These are miscellaneous packages on which sometimes much better than pre-package tools.
fill-column-indicator
– instantly puts a line indicator specifying an 80th column (can configured to be different).gist
– can put a buffer or region into the github gist instantly.ag
– much more faster than grep.fzf
– a fast fuzzy finder.
(use-package fill-column-indicator
:ensure t
:defer 5)
(use-package gist
:ensure t)
(use-package ag
:ensure t)
(use-package fzf
:ensure t)
Rust
The rust
package is still an early version and can’t considered be complete. But we will include it, as such its useful for syntax highlight and minor auto completion.
(use-package rust-mode
:defer 1)
(use-package racer
:diminish racer-mode
:after (rust-mode company eldoc)
:init
(setq racer-rust-src-path
(concat (getenv "HOME")
"/.rustup/toolchains/nightly-x86_64-unknown-linux-gnu/lib/rustlib/src/rust/src"))
(setq racer-cmd (concat (getenv "HOME") "/.cargo/bin/racer"))
:hook ((rust-mode . racer-mode)
(racer-mode . company-mode)
(racer-mode . eldoc-mode)))
(use-package flycheck-rust
:ensure t
:after (rust-mode flycheck)
:hook (flycheck-mode . flycheck-rust-setup))
(use-package cargo
:ensure t
:after (rust-mode)
:hook (rust-mode . cargo-minor-mode))
Typescript
The typescript-mode
is for syntax highlighting while tide
is for LSP1 type analysis and auto completion. Its a good package.
(use-package typescript-mode
:defer 1)
(use-package tide
:ensure t
:diminish tide-mode
:after (typescript-mode company flycheck)
:hook ((typescript-mode . tide-setup)
(typescript-mode . tide-hl-identifier-mode)
(before-save . tide-format-before-save)))
Elixir
The alchemist
package along with elixir-mode
completes Emacs. With its auto-completion to keyword suggestion. I’m just in awe with it, if you’re in elixir programming no one can beat alchemist in it.
(use-package elixir-mode
:defer 1
:hook (before-save . whitespace-cleanup))
(use-package alchemist
:defer 1
:after (elixir-mode))
(use-package web-mode
:defer 1
:mode ("\\.eex\\'" . web-mode)
:config
(setq web-mode-markup-indent-offset 2
web-mode-css-indent-offset 2
web-mode-code-indent-offset 2))
Emmet
The emmet
package similar to other implementaion in other text editor, will auto expand abbreviated tags. Its more combination to web-mode, css-mode and html-mode.
(use-package emmet-mode
:defer 1
:hook ((sgml-mode . emmet-mode)
(web-mode . emmet-mode)
(css-mode . emmet-mode))
:config (progn
(setq emmet-move-cursor-between-quotes t)))
Dart
The dart-mode
handles linting and sometimes connected to dart-analyzer. For me its still not complete but usable. Can configure to auto completion.
(setq js-indent-level 2)
(use-package js2-mode
:ensure t)
(use-package dart-mode
:defer 1
:config
(progn
(setq dart-sdk-path "/opt/flutter/bin/cache/dart-sdk/")
(setq dart-enable-analysis-server t)))
ASCIIDoctor
The adoc-mode
and writegood-mode
, the former is for syntax highlight and formatting while the latter is for checking complexity and grammar of paragraphs.
(use-package adoc-mode
:ensure t
:mode "\\.adoc\\'")
(use-package writegood-mode
:ensure t
:diminish writegood-mode
:after (adoc-mode)
:hook (text-mode . writegood-mode)
:bind
("C-c g" . writegood-mode)
("C-c C-g g" . writegood-grade-level)
("C-c C-g e" . writegood-reading-ease))
Full source
You know its not that hard. Here is the full source with commentary:
;;; init.el --- Initialization file for Emacs 24
;;; Commentary:
;;; ffimnsr <[email protected]>
;; -*- lexical-binding: t; -*-
(unless (>= emacs-major-version 24)
(error "Emacs version 24 or higher is required"))
;;; Code: Emacs 24 or higher only
;; Hasten up startup
(setq gc-cons-threshold (* 24 1024 1024))
(add-hook 'after-init-hook (lambda ()
(setq gc-cons-threshold (* 8 1024))))
;; Default to UTF-8
(prefer-coding-system 'utf-8)
(set-default-coding-systems 'utf-8)
(set-terminal-coding-system 'utf-8)
(set-keyboard-coding-system 'utf-8)
(setq-default buffer-file-coding-system 'utf-8)
(set-frame-font "-SRC-Hack-normal-normal-normal-*-11-*-*-*-m-0-iso10646-1")
;; Unset C-z that freezes emacs gui
(global-unset-key (kbd "C-z"))
;; Buffers reflect external file chanes
(global-auto-revert-mode t)
;; Load emacs in full screen mode and set the title
(when (display-graphic-p)
(add-to-list 'default-frame-alist '(fullscreen . maximized))
(setq frame-title-format "Equivalent Exchange"))
;; Turn off interface early
(if (fboundp 'menu-bar-mode)
(menu-bar-mode -1))
(if (fboundp 'tool-bar-mode)
(tool-bar-mode -1))
(if (fboundp 'scroll-bar-mode)
(scroll-bar-mode -1))
(setq inhibit-startup-screen t ; Disable startup screen with graphics
inhibit-startup-echo-area-message t ; Disable startup echo messages
visible-bell nil ; Disable visual bell graphics
load-prefer-newer t ; Load newer files
ring-bell-function 'ignore) ; Disable audio bell
(when (eq system-type 'darwin)
(add-to-list 'exec-path "/usr/local/bin")
(setq mac-option-modifier 'super
mac-command-modifier 'meta
mac-function-modifer 'control)
(global-set-key "\M-`" 'other-frame))
(setq ns-function-modifier 'control)
(require 'package)
(setq package-enable-at-startup nil)
(setq package-archives
'(("gnu" . "http://elpa.gnu.org/packages/")
("melpa" . "http://melpa.org/packages/")
("melpa-stable" . "http://stable.melpa.org/packages/")
("org" . "https://orgmode.org/elpa/")
("marmalade" . "http://marmalade-repo.org/packages/")))
(package-initialize)
;; Bootstrap packages
(unless (package-installed-p 'use-package)
(package-refresh-contents)
(package-install 'use-package))
(eval-when-compile
(require 'use-package))
(use-package diminish)
(use-package bind-key)
(use-package org
:ensure org-plus-contrib
:defer 7)
(global-set-key (kbd "C-6") 'mode-line-other-buffer)
(setq-default use-package-always-defer t
use-package-always-ensure t
indent-tabs-mode nil ; Use spaces instead of tabs
tab-width 2) ; Set tab width as four spaces is a tab
;; Highlight paired parenthesis
(show-paren-mode t)
;; Enable moving to different windows using meta
(windmove-default-keybindings 'meta)
;; Disable yes-or-no
(fset 'yes-or-no-p 'y-or-n-p)
;; Enable window undo and redo using C-c <left>
(when (fboundp 'winner-mode)
(winner-mode 1))
(setq make-backup-files nil ; Disable backup files
auto-save-default nil
confirm-nonexistent-file-or-buffer nil ; Disable annoying confirmation for not exist
confirm-kill-emacs 'yes-or-no-p ; Confirm before ending emacs session
compile-command "make" ; Set the default compile command
history-delete-duplicates t ; Delete duplicates in minibuffer history
compilation-read-command nil ; Disable confirmation of compile command
epg-gpg-program "/usr/local/bin/gpg") ; Set GPG binary
;; Flatten the mode-line so it would not look like a button
(set-face-attribute 'mode-line nil :box nil)
(set-face-attribute 'mode-line-inactive nil :box nil)
(set-face-attribute 'mode-line-highlight nil :box nil)
;; Configure dired
(setq dired-dwim-target t
dired-recursive-deletes t
dired-use-ls-dired nil
delete-by-moving-to-trash t)
;; Load monokai theme
(when (display-graphic-p)
(load-theme 'monokai t))
;; Make buffer names unique
(require 'uniquify)
(setq uniquify-buffer-name-style 'forward)
(use-package exec-path-from-shell)
(when (memq window-system '(mac ns))
(exec-path-from-shell-initialize)
(exec-path-from-shell-copy-env "GOPATH"))
(use-package flycheck
:defer 5
:diminish flycheck-mode
:hook (after-init . global-flycheck-mode)
:config
(flycheck-define-checker proselint
"A linter for prose."
:command ("proselint" source-inplace)
:error-patterns
((warning line-start (file-name) ":" line ":" column ": "
(id (one-or-more (not (any " "))))
(message) line-end))
:modes (text-mode markdown-mode adoc-mode gfm-mode org-mode))
(add-to-list 'flycheck-checkers 'proselint))
(use-package magit
:pin melpa-stable
:defer 1
:init (diminish 'magit-auto-revert-mode)
:hook (magit-status-sections . magit-insert-worktrees)
:config (progn
(setq magit-commit-show-diff nil
magit-last-seen-setup-instructions "1.4.0")))
(use-package multiple-cursors
:bind ("C-S-c C-S-c" . mc/edit-lines))
(use-package eldoc
:init (diminish 'eldoc-mode))
(use-package expand-region
:bind ("C-=" . er/expand-region))
(use-package helm
:bind (("M-x" . helm-M-x)
("C-x C-f" . helm-find-files))
:diminish helm-mode
:config (progn
(setq helm-buffers-fuzzy-matching t)
(setq helm-grep-ag-command "rg --color=always --colors 'match:fg:black' --colors 'match:bg:yellow' --smart-case --no-heading --line-number %s %s %s")
(setq helm-grep-ag-pipe-cmd-switches '("--colors 'match:fg:black'" "--colors 'matcnh:bg:yellow'"))
(helm-mode 1)))
(use-package projectile
:diminish projectile-mode
:config (progn
(projectile-mode +1)
(setq projectile-enable-caching nil)
(setq projectile-completion-system 'helm)))
(use-package helm-projectile
:bind ("M-t" . helm-projectile-find-file)
:config
(helm-projectile-on))
(use-package company
:defer 1
:diminish company-mode
:init (global-company-mode)
:config
(progn
(global-set-key "\M-n" 'company-select-next)
(global-set-key "\M-p" 'company-select-previous)))
(use-package yasnippet
:ensure t
:diminish yas-minor-mode
:init (yas-global-mode 1))
(use-package yasnippet-snippets
:defer 1
:after (yasnippet))
(use-package which-key
:defer 1
:diminish which-key-mode
:config
(which-key-mode))
(use-package reveal-in-osx-finder)
(use-package ansi-color
:init
(defun m/colorize-compilation-buffer ()
(when (eq major-mode 'compilation-mode)
(ansi-color-apply-on-region compilation-filter-start (point-max))))
:hook (compilation-filter . m/colorize-compilation-buffer))
(use-package yaml-mode)
(use-package markdown-mode
:hook (markdown-mode . visual-line-mode))
(use-package editorconfig
:config
(editorconfig-mode 1))
(use-package rainbow-delimiters
:hook (prog-mode . rainbow-delimiters-mode))
(use-package vlf)
(use-package rust-mode
:defer 1)
(use-package racer
:diminish racer-mode
:after (rust-mode company eldoc)
:init
(setq racer-rust-src-path
(concat (getenv "HOME")
"/.rustup/toolchains/nightly-x86_64-unknown-linux-gnu/lib/rustlib/src/rust/src"))
(setq racer-cmd (concat (getenv "HOME") "/.cargo/bin/racer"))
:hook ((rust-mode . racer-mode)
(racer-mode . company-mode)
(racer-mode . eldoc-mode)))
(use-package flycheck-rust
:ensure t
:after (rust-mode flycheck)
:hook (flycheck-mode . flycheck-rust-setup))
(use-package cargo
:ensure t
:after (rust-mode)
:hook (rust-mode . cargo-minor-mode))
(use-package typescript-mode
:defer 1)
(use-package tide
:ensure t
:diminish tide-mode
:after (typescript-mode company flycheck)
:hook ((typescript-mode . tide-setup)
(typescript-mode . tide-hl-identifier-mode)
(before-save . tide-format-before-save)))
(use-package toml-mode
:defer 1)
(use-package solidity-mode
:ensure t
:no-require t)
(use-package company-solidity
:ensure t
:no-require t
:config (add-hook 'solidity-mode-hook
(lambda ()
(my-company-add-backend-locally 'company-solidity))))
(use-package elixir-mode
:defer 1
:hook (before-save . whitespace-cleanup))
(use-package alchemist
:defer 1
:after (elixir-mode))
(use-package web-mode
:defer 1
:mode ("\\.eex\\'" . web-mode)
:config
(setq web-mode-markup-indent-offset 2
web-mode-css-indent-offset 2
web-mode-code-indent-offset 2))
(use-package emmet-mode
:defer 1
:hook ((sgml-mode . emmet-mode)
(web-mode . emmet-mode)
(css-mode . emmet-mode))
:config (progn
(setq emmet-move-cursor-between-quotes t)))
(use-package cc-mode
:hook ((c-mode . (lambda () (c-set-style "bsd")))
(java-mode . (lambda () (c-set-style "bsd"))))
:config
(progn
(setq tab-width 2)
(setq c-basic-offset 2)))
(setq js-indent-level 2)
(use-package js2-mode
:ensure t)
(use-package dart-mode
:defer 1
:config
(progn
(setq dart-sdk-path "/opt/flutter/bin/cache/dart-sdk/")
(setq dart-enable-analysis-server t)))
(use-package undo-tree
:ensure t
:init
(global-undo-tree-mode))
(use-package adoc-mode
:ensure t
:mode "\\.adoc\\'")
(use-package writegood-mode
:ensure t
:diminish writegood-mode
:after (adoc-mode)
:hook (text-mode . writegood-mode)
:bind
("C-c g" . writegood-mode)
("C-c C-g g" . writegood-grade-level)
("C-c C-g e" . writegood-reading-ease))
(use-package avy
:ensure t
:bind
("H-SPC" . avy-goto-char-timer)
("H-w" . avy-goto-word-1)
("H-c" . avy-goto-char-2)
("H-l" . avy-goto-line)
("H-d" . avy-goto-word-0)
("<f9> SPC" . avy-goto-char-timer)
("C-c g" . avy-goto-word-1)
("M-g l" . avy-goto-line)
("M-g c" . avy-goto-char-2)
("M-g w" . avy-goto-word-0))
(use-package smartparens
:ensure t
:diminish smartparens-mode
:config
(progn
(use-package smartparens-config)
(smartparens-global-mode 1)))
(use-package fill-column-indicator
:ensure t
:defer 5)
(use-package gist
:ensure t)
(use-package ag
:ensure t)
(use-package fzf
:ensure t)
(garbage-collect)
(custom-set-variables
;; custom-set-variables was added by Custom.
;; If you edit it by hand, you could mess it up, so be careful.
;; Your init file should contain only one such instance.
;; If there is more than one, they won't work right.
'(custom-safe-themes
(quote
("c3d4af771cbe0501d5a865656802788a9a0ff9cf10a7df704ec8b8ef69017c68" default)))
'(org-latex-caption-above nil)
'(package-selected-packages
(quote
(gist avy writegood-mode adoc-mode solidity-mode htmlize alchemist web-mode org-plus-contrib typescript-mode racer magit fill-column-indicator markdown-mode vlf monokai-theme diminish emmet-mode rainbow-delimiters reveal-in-osx-finder which-key company helm-config helm-projectile helm projectile flycheck use-package))))
(custom-set-faces
;; custom-set-faces was added by Custom.
;; If you edit it by hand, you could mess it up, so be careful.
;; Your init file should contain only one such instance.
;; If there is more than one, they won't work right.
)
(put 'erase-buffer 'disabled nil)
;;; init.el ends here
Conclusion
We are just exploring the tip of the ice berg. You can add and configure Emacs to your likeness, checkout full configurations like Spacemacs2 if you want a pre-built config. Follow me at @ffimnsr to get new articles and tips.
- The Language Server Protocol is an open, JSON-RPC-based protocol for use between source code editors or integrated development environments and servers that provide programming language-specific features. ↩︎
- Spacemacs is a configuration framework for GNU Emacs. It can take advantage of all of GNU Emacs’ features, including both graphical and command-line user interfaces, and being executable under X Window System and within a Unix shell terminal. ↩︎