Gimp Automating Image Processing with Python Fu
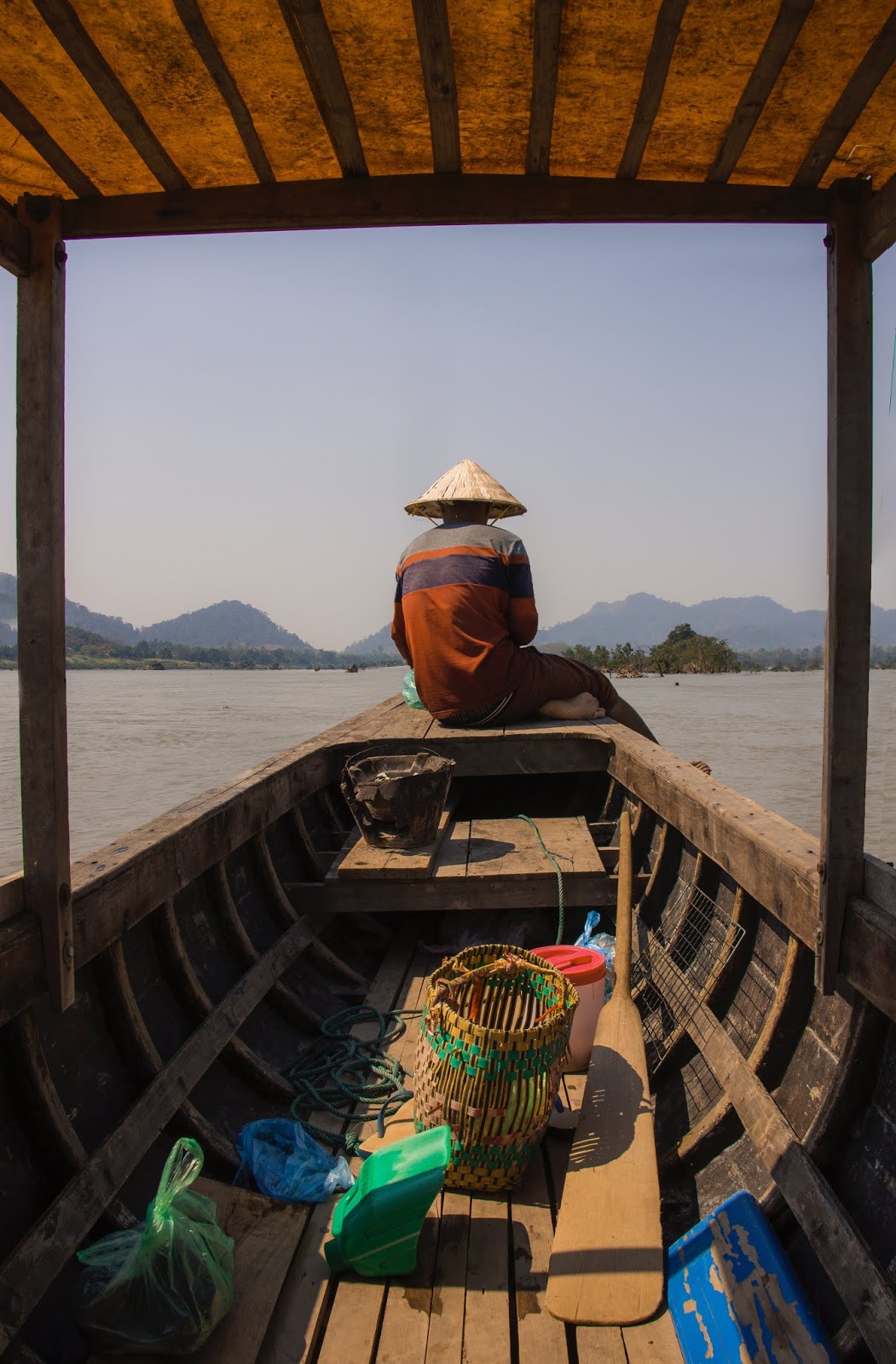
What a large volume of adventures may be grasped within the span of his little life by him who interests his heart in everything.
ā Laurence Sterne.
Hi guys, one night I decided to create an online store and sell some drop ship products. I grab some pictures from the wholesale seller and planned to customize those images (just to put some store branding).
There were a hundred images that I want to customize, by hand it would take ages so I decided to create a batch script. My first thought was to use Gimp (an open source image manipulator) and Script-Fu. After trying out what the result would be on Python-Fu console, I settled with this. It was a simple design but I was satisfied.
Here is the script that I use. Try it out on Python-Fu console and call it convert_to_poster(n)
, where n
is the image number in the image list inside Gimp. You could for loop it for faster batch processing.
First we set the variables.
current_image = gimp.image_list()[n]
center_x = current_image.width / 2
center_y = current_image.height / 2
c_size = 600
c_size_d = c_size / 2
Then we resize the image / canvas.
pdb.gimp_image_resize(current_image, c_size, c_size, c_size_d - center_x, c_size_d - center_y)
We create an empty background layer and just fill it with white.
bg_layer = pdb.gimp_layer_new(current_image, c_size, c_size, RGBA_IMAGE, "bg", 100, LAYER_MODE_NORMAL)
pdb.gimp_drawable_fill(bg_layer, FILL_WHITE)
pdb.gimp_image_add_layer(current_image, bg_layer, 1)
We will also set the main background and foreground color that would be use in future instruction set.
pdb.gimp_context_set_foreground("#960acc")
pdb.gimp_context_set_background("#000000")
Create branding layer and fill the rectangular region with indigo.
branding_layer = pdb.gimp_layer_new(current_image, c_size, c_size, RGBA_IMAGE, "branding", 100, LAYER_MODE_NORMAL)
pdb.gimp_image_add_layer(current_image, branding_layer, 1)
pdb.gimp_selection_none(current_image)
pdb.gimp_image_select_rectangle(current_image, CHANNEL_OP_ADD, 0, 0, c_size, 260)
pdb.gimp_drawable_edit_fill(branding_layer, FILL_FOREGROUND)
Get poster image and resize its layer to fit canvas.
poster_layer = current_image.layers[0]
pdb.gimp_layer_add_alpha(poster_layer)
pdb.gimp_layer_resize_to_image_size(poster_layer)
Select non-alpha on poster layer and grow.
pdb.gimp_image_select_item(current_image, CHANNEL_OP_REPLACE, poster_layer)
pdb.gimp_selection_grow(current_image, 3)
Create a mask and fill it with the background color that we set.
mask = pdb.gimp_layer_create_mask(branding_layer, ADD_MASK_WHITE)
pdb.gimp_layer_add_mask(branding_layer, mask)
pdb.gimp_layer_set_edit_mask(branding_layer, 1)
pdb.gimp_drawable_edit_fill(mask, FILL_BACKGROUND)
Add text node with color it white.
pdb.gimp_context_set_foreground("#ffffff")
text_layer = pdb.gimp_text_fontname(current_image, None, 10.0, 10.0, "Psyche Digital", 0, 1, 24.0, PIXELS, "SF Compact Display Heavy")
We select the main layer and add a legacy drop shadow.
pdb.gimp_image_select_item(current_image, CHANNEL_OP_REPLACE, poster_layer)
pdb.script_fu_drop_shadow(current_image, poster_layer, 10.0, 10.0, 10.0, (0, 0, 0, 255), 80, 0)
Here is the full script code:
def convert_to_poster(n):
current_image = gimp.image_list()[n]
center_x = current_image.width / 2
center_y = current_image.height / 2
c_size = 600
c_size_d = c_size / 2
pdb.gimp_image_resize(current_image, c_size, c_size, c_size_d - center_x, c_size_d - center_y)
bg_layer = pdb.gimp_layer_new(current_image, c_size, c_size, RGBA_IMAGE, "bg", 100, LAYER_MODE_NORMAL)
pdb.gimp_drawable_fill(bg_layer, FILL_WHITE)
pdb.gimp_image_add_layer(current_image, bg_layer, 1)
pdb.gimp_context_set_foreground("#960acc")
pdb.gimp_context_set_background("#000000")
branding_layer = pdb.gimp_layer_new(current_image, c_size, c_size, RGBA_IMAGE, "branding", 100, LAYER_MODE_NORMAL)
pdb.gimp_image_add_layer(current_image, branding_layer, 1)
pdb.gimp_selection_none(current_image)
pdb.gimp_image_select_rectangle(current_image, CHANNEL_OP_ADD, 0, 0, c_size, 260)
pdb.gimp_drawable_edit_fill(branding_layer, FILL_FOREGROUND)
poster_layer = current_image.layers[0]
pdb.gimp_layer_add_alpha(poster_layer)
pdb.gimp_layer_resize_to_image_size(poster_layer)
pdb.gimp_image_select_item(current_image, CHANNEL_OP_REPLACE, poster_layer)
pdb.gimp_selection_grow(current_image, 3)
mask = pdb.gimp_layer_create_mask(branding_layer, ADD_MASK_WHITE)
pdb.gimp_layer_add_mask(branding_layer, mask)
pdb.gimp_layer_set_edit_mask(branding_layer, 1)
pdb.gimp_drawable_edit_fill(mask, FILL_BACKGROUND)
pdb.gimp_context_set_foreground("#ffffff")
text_layer = pdb.gimp_text_fontname(current_image, None, 10.0, 10.0, "Psyche Digital", 0, 1, 24.0, PIXELS, "SF Compact Display Heavy")
pdb.gimp_image_select_item(current_image, CHANNEL_OP_REPLACE, poster_layer)
pdb.script_fu_drop_shadow(current_image, poster_layer, 10.0, 10.0, 10.0, (0, 0, 0, 255), 80, 0)
Run this code in Gimp console or in Script-Fu console.
š„°šššš
Leave a like and subscribe for more hacks, tips, and tricks.