Solidity Series - Building Your First Hello World
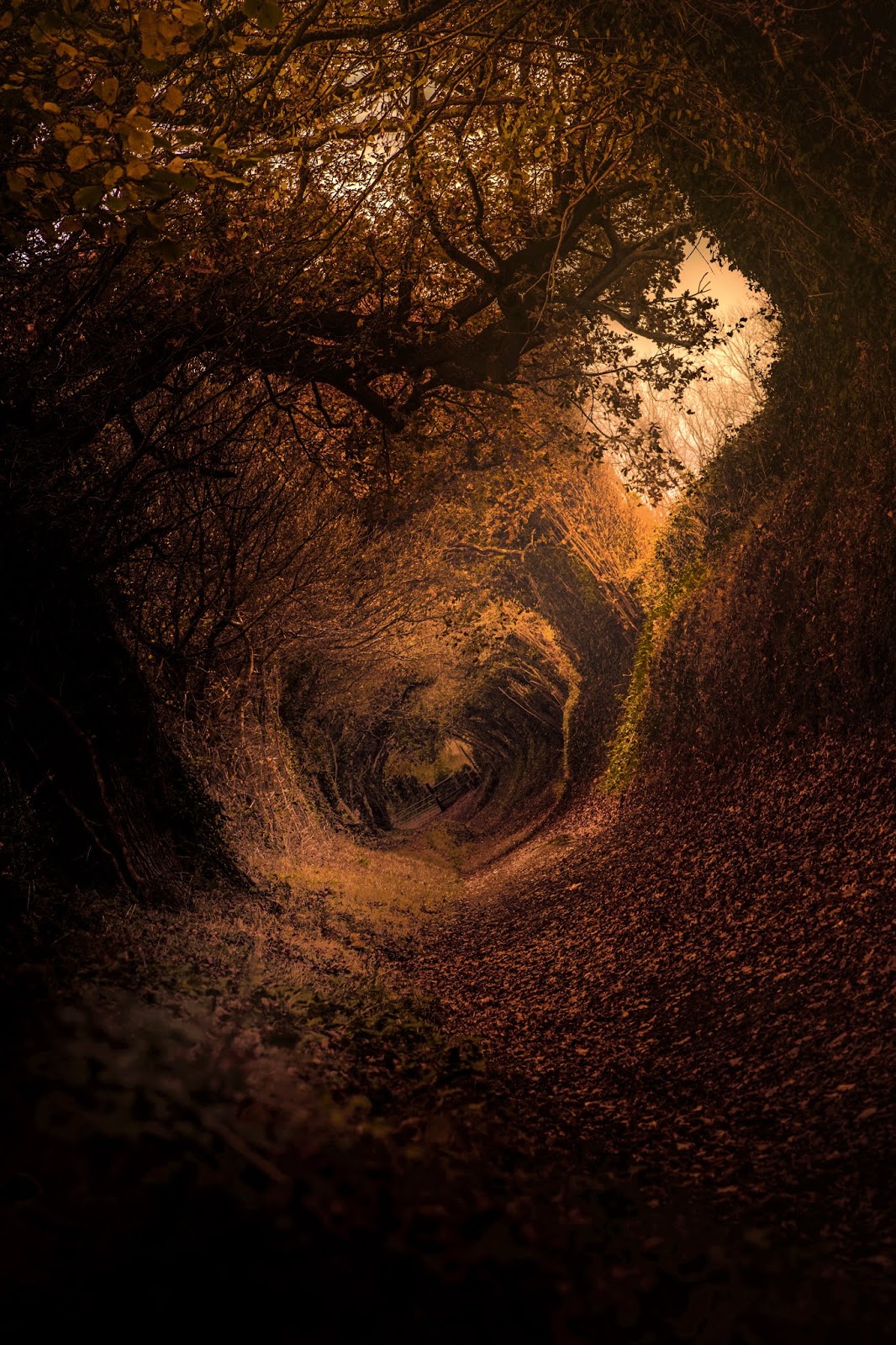
Knowing others is wisdom, knowing yourself is enlightenment.
— Lao Tzu.
It’s the start of decentralization age but how to start programming on decentralize environment?
Ethereum1, considered as second wave of blockchain innovation. The pioneer and first blockchain ecosystem to implement and handle smart contracts. So how do you start developing system and application DApps (Decentralize Apps) in it?
The answer is “Solidity” — a programming language that interface EVM (Ethereum Virtual Machine) and your app.
Prerequisites
In this tutorial we assume you have some basic knowledge in programming.
The main requirements in developing a blockchain app is first its compiler then the secondary requirements but optional are the bootstrap framework like truffle.
- Node 10 and above
Building Hello World
To start the development, we must get the required dependency in developing a structured smart contract project. The tool and framework that we will be using is truffle, which handles all the bootstrapping procedure and creates the base of your project. Installing it is just breeze, all you have to do is type npm install -g truffle
.
Also install ganache
which is the old ethereum-test-rpc
project. To install ganache you have an option whether to install it on a desktop or a CLI (Command Line Interface) build. For this project I’ll be using the CLI build, so to start we install ganache using the command npm install -g ganache-cli
. Both truffle and ganache is under the truffle software development platform.
And now we start building the project, after all dependencies installed we need to create an empty project directory with the command truffle init
. As a note, the init
sub-command only works on an empty directory. This sub-command will create a base structure for our project.
Project Structure
Below is a structure that I recommend to bootstrap your project.
contracts
: Storage of smart contracts source.migrations
: Migration script to deploy smart contract.test
: Test directory for smart contract.truffle-config.js
: Truffle configuration for Windows users (for backward compatibility).truffle.js
: Truffle configuration for *nix system.
To test if everything’s okay we must run truffle compile
.
Running a test RPC for Ethereum
So in order to start, we first need to generate test accounts and run a test environment. Start by typing ganache-cli
in a separate non-occupied terminal window. The tool ganache will generates 10 ETH addresses with an initial balance of 100 ether.
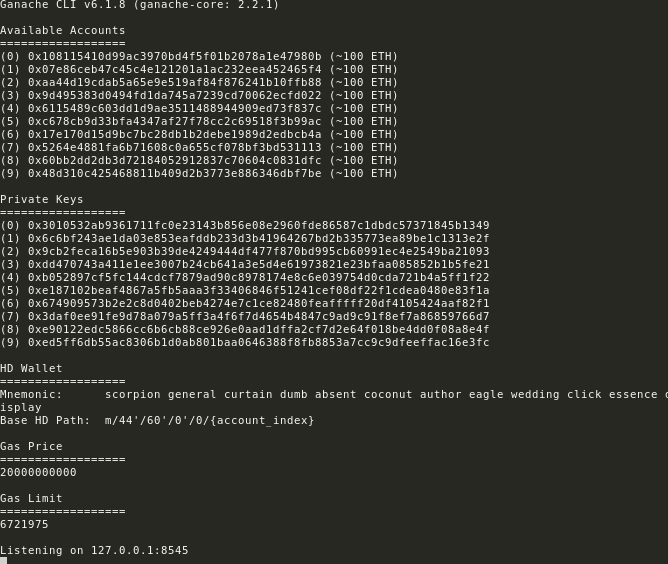
Coding your first hello
With ganache-cli running at background, we continue building our hello world project. To start, we must create a new contract using truffle create contract Hello
on which this command will create a new file under contracts ⇒ Hello.sol. Edit the newly created file and add a new function that will return the string Hello in EVM.
pragma solidity ^0.4.24;
contract Hello {
function greeter() public pure returns (string) {
return "Hello World";
}
}
In the next step, will be creating and editing the migration file. To create the file execute touch migrations/2_deploy_contracts.js
, then edit the new file and link that to the file we created earlier Hello.sol. This is a very crucial step in order to deploy our Hello solidity file on test blockchain environment.
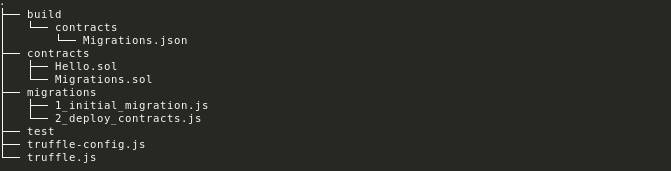
Here are the changes we need in migrations/2_deploy_contracts.js
.
var hello = artifacts.require("./Hello.sol");
module.exports = function(deployer) {
deployer.deploy(hello);
}
After we edit the migration file, we can now connect the truffle project to ganache test blockchain RPC environment. To do that we’ll be editing truffle.js
and point it to ganache host and port which is localhost:8545
.
module.exports = {
networks: {
development: {
host: "localhost",
port: 8545,
},
},
};
When everything’s done, execute truffle migrate
. This command will automatically compile and deploy the smart contract into ganache test blockchain RPC environment. Remember and copy the Hello function address in the output console, we will be using this later.
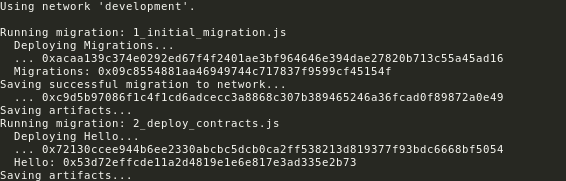
To test the function if its already deployed in the test environment, we will execute a REPL console using truffle console
. Then inside the console, we type Hello.at(address).greeter()
(note the address here is the one we copy earlier).

If everything is correct it should output Hello World
. That’s it, now you’re on your way to become a blockchain developer.This is the link to the whole source code.
Conclusion
Hey guys, now that you’ve finish the setup you are now on the path of becoming a blockchain software developer. Follow us on our next adventure developing deep into the blockchain ecosystem. Stay tuned for the next follow up series for solidity.
- Ethereum is an open source, public, blockchain-based distributed computing platform and operating system featuring smart contract functionality. It supports a modified version of Nakamoto consensus via transaction-based state transitions. ↩︎