Flutter Series - Creating Your First Hello World
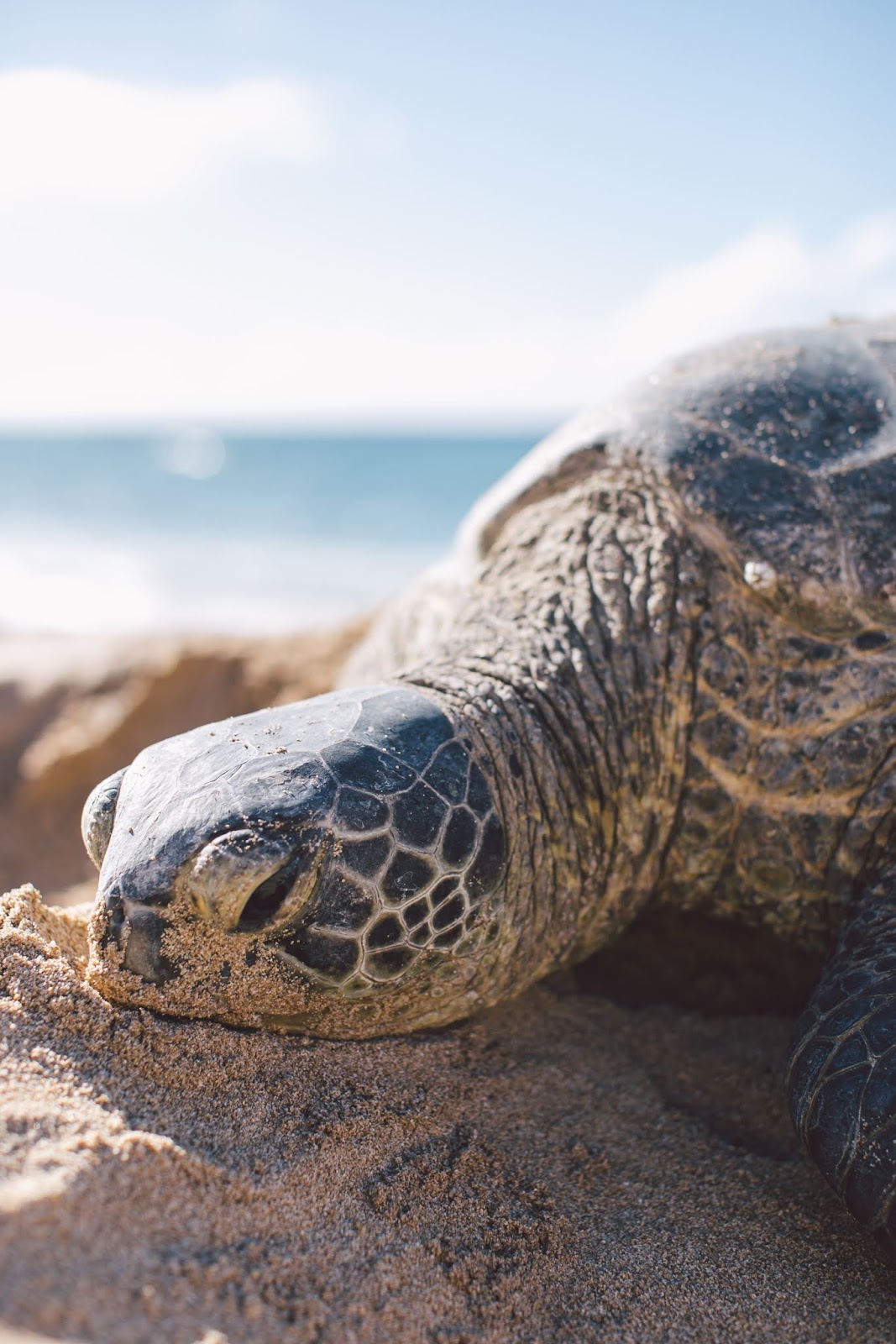
When you can’t find the sunshine, be the sunshine!
— Anonymous.
Have you ever dreamt of building your own app but think it's too hard? Why don’t you try flutter…
Flutter is a new way to build multi-platform apps which is currently backed and developed by Google. It's often compared to react native, but the difference is performance. React Native runs on JavaScript bridge while Flutter on dart bridge. What dart bridge does best is AOT (ahead of time) compiling to native ARM code.
Prerequisites
First, you need flutter, just follow the steps below to install it.
- Flutter 1.0
Building Hello World
First, we will install flutter, depending on your operating system you can find the details on how to install flutter here. On arch Linux you can find it on AUR repository.
After installing the flutter package. Run flutter doctor
to check for any dependency error.
And now we begin, to start a flutter project you need to type in flutter create <project-name>
. For example, I’m building a Hello World project named stocks
.
flutter create stocks
The command will create a directory named stocks
which contains minimal running app template. What we do first is open the file lib/main.dart
using your favorite text editor and rename the app title Flutter Demo
to Hello World
. Then remove the whole Scaffold
block as we will create our own scaffold. After the changes your _MyHomePageState
class would look like this.
// ....
class _MyHomePageState extends State<MyHomePage> {
int _counter = 0;
void _incrementCounter() {
setState(() {
_counter++;
});
}
@override
Widget build(BuildContext context) {
return Scaffold();
}
}
// ....
Clean up the unused functions and variables like _counter
and _incrementCounter
. If your editor has integrated linter and dart analyzer, it would complain regarding unused variable. Once cleanup’s done, implement now a centered hello world text by adding body to the scaffold.
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Text('Hello World!'),
),
);
}
}
Now run your code using the command flutter run
. If a device’s connected it will show an app displaying a centered hello world. You could also use an emulator by running flutter launch
before running flutter run
.
So, what’s next?
Now after everything’s done, we will continue building our stocks monitor app in the next chapter. Stay tuned.